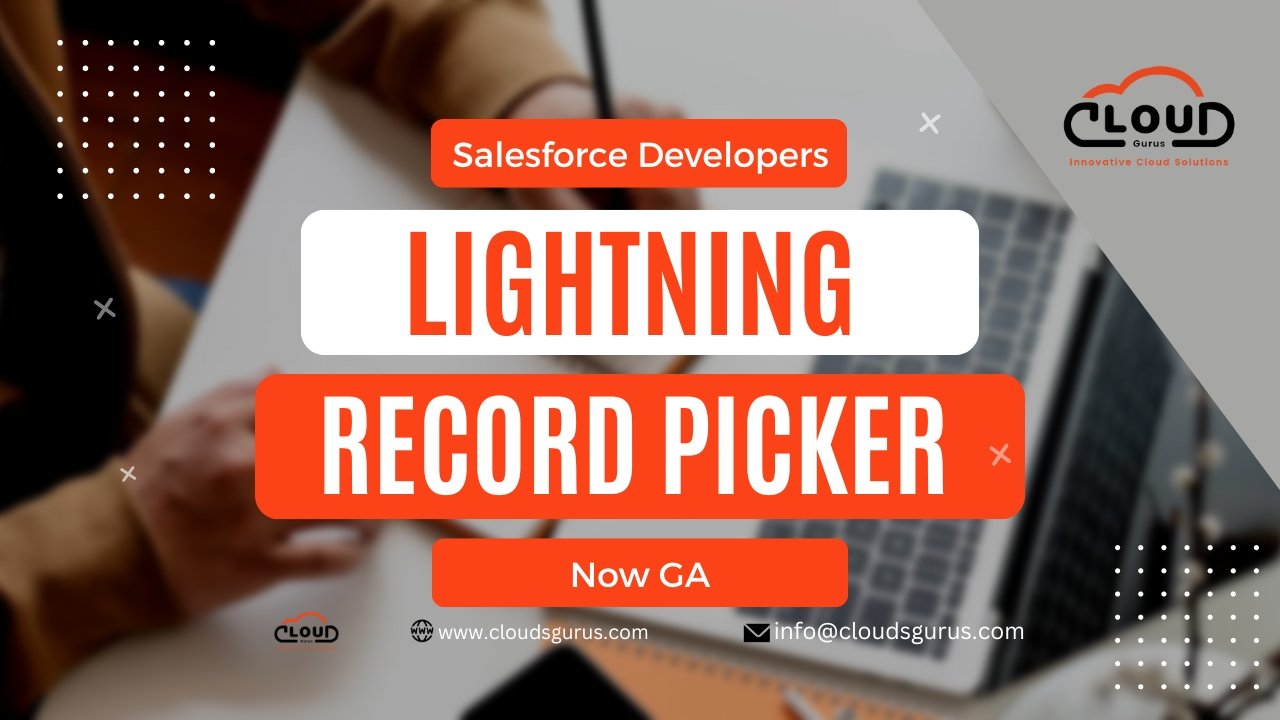
Lightning Record Picker Now Generally Available
Have you ever struggled to build your own custom component for finding and selecting Salesforce records?
But now the Lightning Record Picker is generally available in Spring ’24, bringing a powerful and flexible LWC Component for Salesforce developers. In this post, we’ll explore what the Lightning Record Picker is, its benefits, and how to use it with practical code examples
What is the Lightning Record Picker?
The lightning-record-picker
component allows you to search for a list of Salesforce Records that match search input. It uses the GraphQL wire adapter to search for records, displays the records, and allows you to select a record.
Implementing the Lightning Record Picker Examples:
Basic Example
The following example demonstrates a basic implementation of the Lightning Record Picker to search for Account objects.
HTML File (recordPickerBasic.html):
<template>
<lightning-card title="Record Picker Example" icon-name="custom:custom63">
<div class="slds-m-around_medium">
<lightning-record-picker
label="Accounts"
placeholder="Search Accounts..."
object-api-name="Account"
>
</lightning-record-picker>
</div>
</lightning-card>
</template>
Advanced Customization
You can specify filters to customize which records are displayed in the record picker. Below is an example of how to apply filters.
The value for filter is an object with a criteria property and an optional filterLogic property. The value for criteria is an array with a list of filter criteria objects.
Below example filters records where the Website field equals “https://www.cloudsgurus.com” or null, the Name of the related Parent start with “Cloud”, and Type equal “Partner”.
HTML File (recordPickerWithFilters.html):
<template>
<lightning-card title="Filtered Record Picker" icon-name="custom:custom63">
<div class="slds-m-around_medium">
<lightning-record-picker
object-api-name="Account"
label="Accounts"
filter={filter}>
</lightning-record-picker>
</div>
</lightning-card>
</template>

HTML File (recordPickerWithFilters.js):
import { LightningElement, track } from 'lwc';
export default class RecordPickerWithFilters extends LightningElement {
@track filter = {
criteria: [
{
fieldPath: 'Website',
operator: 'eq',
value: 'https://www.cloudgurus.com',
},
{
fieldPath: 'Website',
operator: 'eq',
value: null,
},
{
fieldPath: 'Type',
operator: 'eq',
value: 'Partner',
},
{
fieldPath: 'Parent.Name',
operator: 'like',
value: 'Cloud%',
},
],
filterLogic: '(1 OR 2) AND 4 AND 3',
};
}
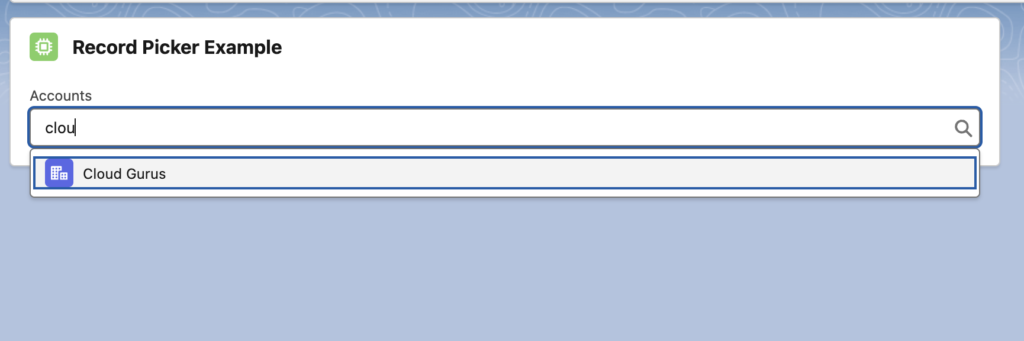
Customizing Display of Record Suggestions:
You can customize how record suggestions appear by using the display-info
attribute. This attribute specifies the main field and additional fields to display in the record picker results.
This example sets the primary field to the name of the related account and adds Title
as a display field in the returned record picker results.
displayInfo = {
primaryField: 'Account.Name',
additionalFields: ['Title'],
};
<lightning-record-picker
object-api-name="Contact"
label="Contacts"
display-info={displayInfo}>
</lightning-record-picker>
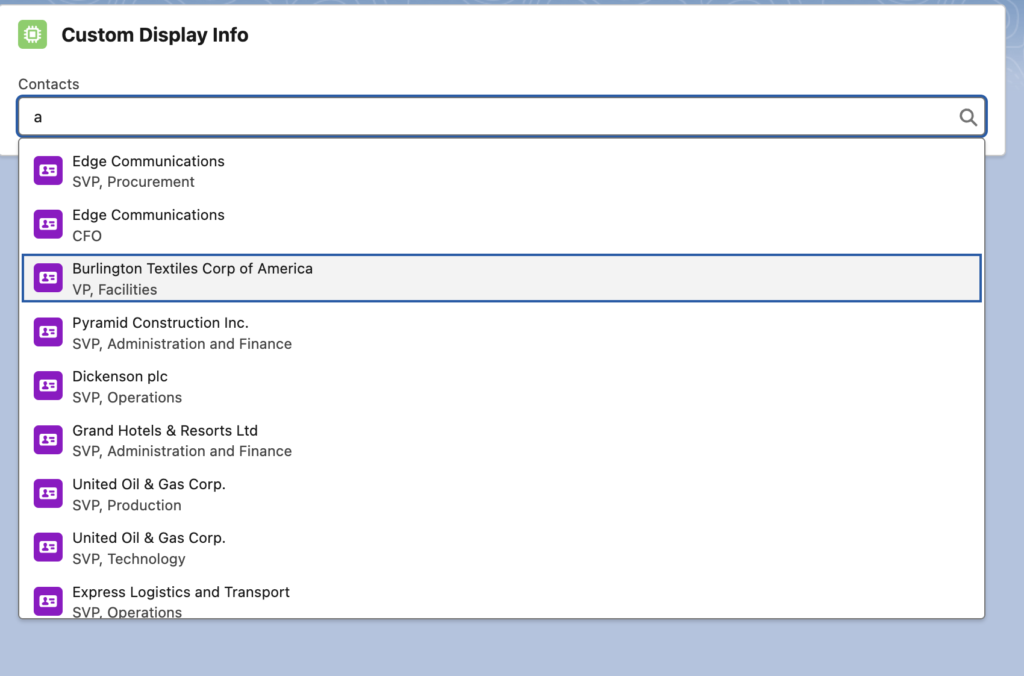
Defining Filter Logic and Criteria
To define logic for the set of filter criteria, use the filterLogic
property. If filterLogic
is undefined, all criteria are applied with an AND logical operator by default. The valid operators are AND, OR, and NOT.
Filter Criteria Object
The filter
object requires the following properties for each element in the criteria array:
- fieldPath (required): The API name of the field to filter on.
- operator (required): The filter operator.
- value (required): The value to filter against.
Error Handling and Events
The Lightning Record Picker can return both blocking and non-blocking errors. It also fires several custom events, such as change
, error
, focus
, blur
, and ready
, to handle various interactions and states.
Conclusion
The Lightning Record Picker is a powerful and customizable tool that enhances your Salesforce experience by making record selection easier and more efficient. By following the examples provided, you can quickly implement and customize this component to meet your specific needs.